There are various type of thresholding. We will discuss one by one:
Simple Thresholding
If pixel value less then threshold value then it is assigned one value else we will assign another value. For thresholding we will use cv2.threshold function.
In this function we will pass four parameters:
1: Source image: Source image should be gray scale image.
2: Threshold value: It is used classify the pixel value
3: maxVal: Maximum value that can be assign to a pixel.
4: Thresholding technique: Apply any type of thresholding (cv2.THRESH_BINARY, cv2.THRESH_BINARY_INV, cv2.THRESH_TRUNC, cv2.THRESH_TOZERO, cv2.THRESH_TOZERO_INV)
Simple Thresholding Techniques
- cv2.THRESH_BINARY: If pixel intensity is greater than the set threshold, value set to 255, else set to 0 (black).
- cv2.THRESH_BINARY_INV: Inverted case of cv2.THRESH_BINARY.
- cv2.THRESH_TRUNC: If pixel intensity value is greater than threshold, it is truncated to the threshold. The pixel values are set to be the same as the threshold. All other values remain the same.
- cv2.THRESH_TOZERO: Pixel intensity is set to 0, for all the pixels intensity, less than the threshold value.
- cv2.THRESH_TOZERO_INV: Inverted case of
cv2.THRESH_TOZERO
.
Adaptive Thresholding
In the Adaptive thresholding pixel value calculated for smaller regions, so we can get different threshold for different region. It gives us better result where lighting areas different.
cv.AdaptiveThreshold(src, dst, maxValue, adaptive_method=CV_ADAPTIVE_THRESH_MEAN_C, thresholdType=CV_THRESH_BINARY, blockSize=3, param1=5)
- src: Source Image
- dst: Destination image of the same size and the same type as src.
- maxValue: Max value this we can assign to pixel
- adaptiveMethod: Adaptive thresholding algorithm to use, ADAPTIVE_THRESH_MEAN_C or ADAPTIVE_THRESH_GAUSSIAN_C. This decides how thresholding value is calculated.
- thresholdType – Thresholding type ( THRESH_BINARY or THRESH_BINARY_INV)
- blockSize – size of a pixel neighbourhood that is used to calculate a threshold value for the pixel: 3, 5, 7, and so on.
- Constant: A constant value substracted from the mean or weighted sum of the neighbourhood pixel.
import cv2 as cv
import numpy as np
from matplotlib import pyplot as plt
img = cv.imread('excel.png',0)
img = cv.medianBlur(img,5)
ret,th1 = cv.threshold(img,127,255,cv.THRESH_BINARY)
th2 = cv.adaptiveThreshold(img,255,cv.ADAPTIVE_THRESH_MEAN_C,\
cv.THRESH_BINARY,11,2)
th3 = cv.adaptiveThreshold(img,255,cv.ADAPTIVE_THRESH_GAUSSIAN_C,\
cv.THRESH_BINARY,11,2)
titles = ['Original Image', 'Global Thresholding (v = 127)',
'Adaptive Mean Thresholding', 'Adaptive Gaussian Thresholding']
images = [img, th1, th2, th3]
for i in range(4):
plt.subplot(2,2,i+1),plt.imshow(images[i],'gray')
plt.title(titles[i])
plt.xticks([]),plt.yticks([])
plt.show()
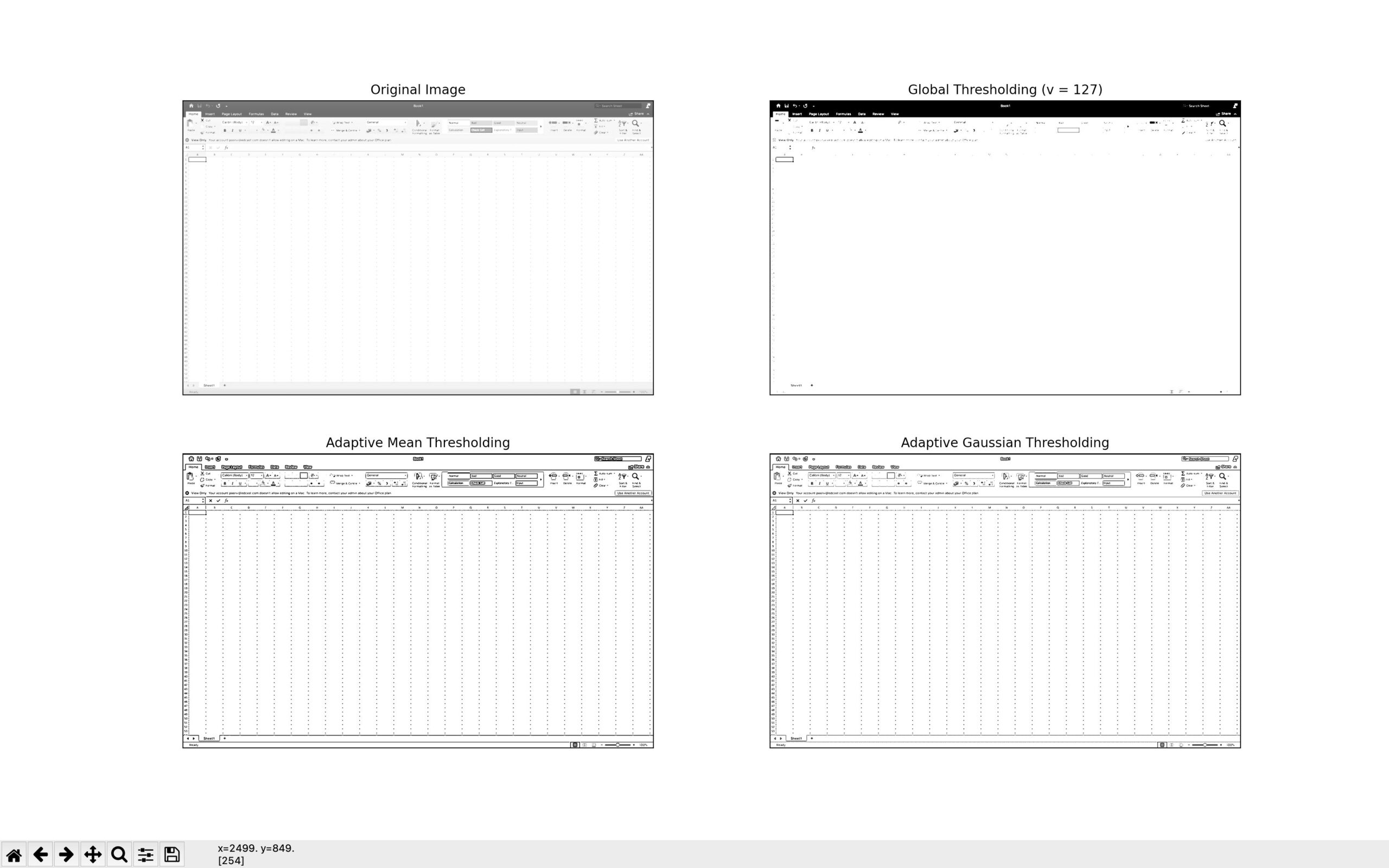
Otsu’s Thresholding
Process of Otsu’s thresholding,
- Process the input image.
- Obtain histogram image.
- Then calculate the threshold value.
- Replace image pixels into white where saturation is greater than threshold and replace in black in opposite cases.