Smoothing or blurring of the most commonly used techniques for image processing. It is used to suppress the noise of the pictures. Linear filters are used for smoothing. The linear filters are simple to use and quick.
There are several kinds of linear filters:
- Homogeneous filter
- Gaussian filter
- Median Filter
- Bilateral Filter
Homogeneous filter
Homogeneous filter is very simple filter, each output pixel is the mean of its kernel neighbours. In homogenous filter each filter compute with common weight, that’s why we called this filter is homogenous filter.
In homogeneous filter we should specify the width and height of kernel. A 3×3 normalized box filter would look like this:
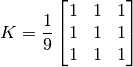
In homogeneous filter we will use filter2D function. filter2D function smooth images and removed noise from image.
cv2.filter2D(img, -1, kernel)
Similar to one-dimensional signals, images can also be filtered with low-pass filters and high-pass filters.
LPF helps in removing noises and blurring the images
HPF filters helps in finding edges in the images.
Blur image function
cv2.blur(img, (5,5))
Gaussian filter
Gaussian filter uses kernel of different weight in x and y direction. Pixel which are located in center they have high weight and pixel which is far from center they have low weight.
Syntax:
cv2.GaussianBlur(img, Kernel, sigmavalue)
cv2.GaussianBlur(img, (5, 5), 0)
Gaussian filter mainly used for remove high frequency noise in images.
Median Filter
In median filter we will replace each pixel value with the median of its neighbouring pixel. This method is used for remove salt and pepper noise.
cv2.medianBlur(img, Kearnel)
Bilateral Filter
The bilateral filter is useful when we need to smooth out pictures and eliminate noise, while keeping the edges clean.
cv.bilateralFilter(img, d, sigmaColor, sigmaColor)
d – Diameter of each pixel neighbourhood
sigmaColor: Filter sigma in the color space.
sigmaColor: Filter sigma in the coordinate space.
import cv2
import numpy as np
from matplotlib import pyplot as plt
img = cv2.imread('redimg.jpg')
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
kernel = np.ones((5, 5), np.float32)/25
dstImg = cv2.filter2D(img, -1, kernel)
blurImg = cv2.blur(img, (5, 5))
gblurImg = cv2.GaussianBlur(img, (5, 5), 0)
medianImg = cv2.medianBlur(img, 5)
bilateralFilterImg = cv2.bilateralFilter(img, 9, 75, 75)
titles = ['image', '2D Convolution', 'blur', 'GaussianBlur', 'median', 'bilateralFilter']
images = [img, dstImg, blurImg, gblurImg, medianImg, bilateralFilterImg]
for i in range(6):
plt.subplot(2, 3, i+1), plt.imshow(images[i], 'gray')
plt.title(titles[i])
plt.xticks([]),plt.yticks([])
plt.show()
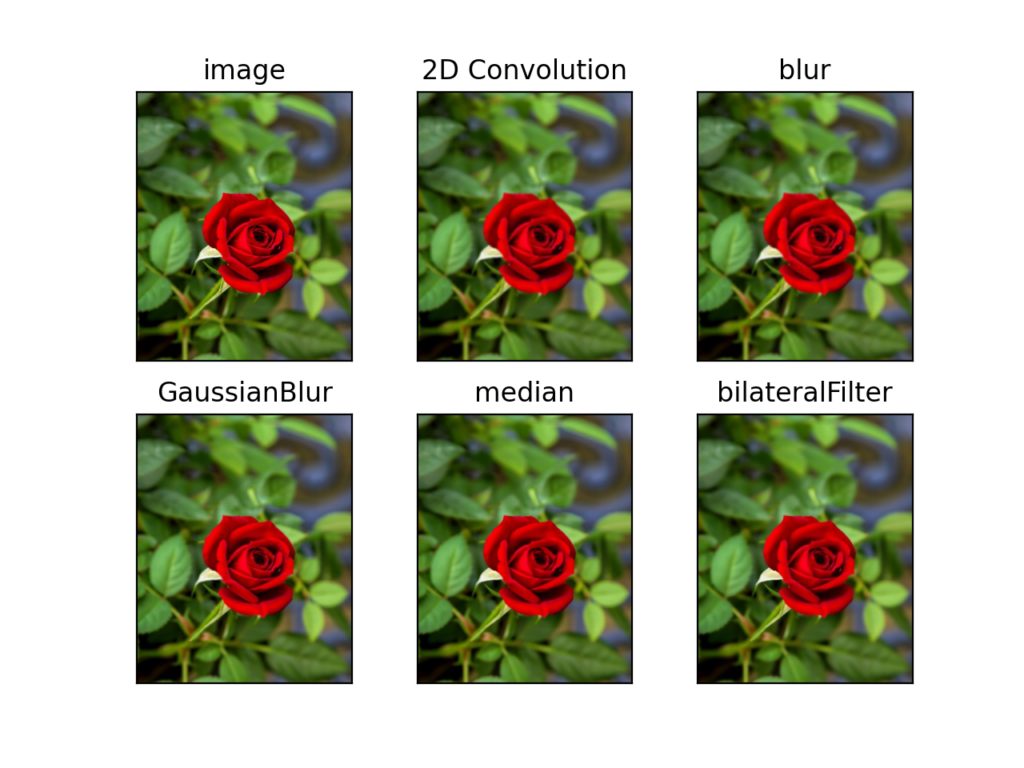